Wolmer’s
INFORMATION TECHNOLOGY (GENERAL PROFICIENCY)
Grade 11 Teacher: Mrs. McCallum-Rodney
File Processing
Introduction
Storage of data in variables and arrays are temporary; all such data is lost when a program terminates. Files are used for permanent retention of large amounts of data. Computers store files on secondary storage mediums. Our focus in this lesson will be on creating, updating and processing data files by C programs.
The Data Hierarchy
Bit - the smallest data item in a computer can assume the value of 0 or the value of 1. Such a data item is called a bit (short for “binary digit” – a digit that can assume one of two values.). Computer circuitry performs various simple bit manipulations such as determining the value of a bit, setting the value of a bit, and reversing a bit (from 1 to 0 and vice versa).
Characters – It is cumbersome for programmers to work with data in the low-level form of bits. Instead, programmers prefer to work with data in the form of decimal digits (0 to 9), letters (A to Z, a to z) and special symbols (such as $, @, %, &, and many others). Digits, letters and symbols are referred to characters. Since computers can process only 1s and 0s, every character in a computer’s character set is represented as a pattern of 1ws and 0s (called a byte – 8 bits make a byte).
Fields – this is a group of characters that conveys a meaning. For example, a field consisting solely of uppercase and lowercase letters can be used to represent a person’s name.
Records – This is a STRUCT in C and is composed of several fields. In a payroll system, for example, a record for a particular employee might consist of the following fields:
- Social Security Number
- Name
- Address
- Hourly salary rate
- Amount of taxes withheld
Thus, a record is a group of related fields. In the preceding example, each of the fields belongs to the same employee. A company may have many employees, and will have a payroll record for each employee.
File – This is group of related records.
Database – most businesses utilize many different files to store data. For example a company may have payroll files, account receivable files, inventory files and many other types of files. A group of related files is sometimes called a database. A collection of programs designed to create and manage databases is called a database management system (DBMS).
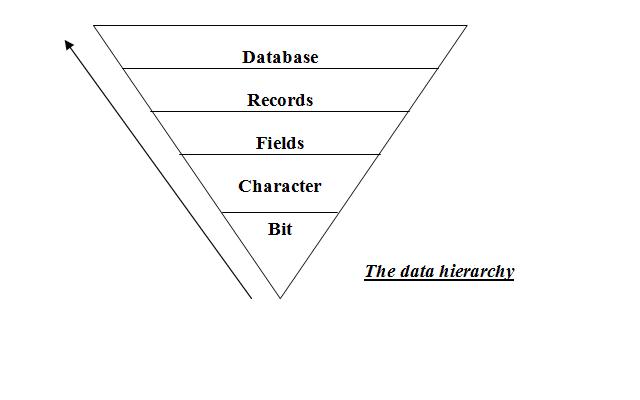
To facilitate the retrieval of specific records form a file, at least one field in each record is chosen as a record key (previously known to you as a primary key). A record key identifies a record as belonging to a particular person or entity. For example, in the payroll record, the Social security number would normally be chosen as the record key.
Sequential File
There are many ways to organize records in a file. The most popular type of organization is called a sequential file in which records are typically stored in order by the record key field.
File processing is traditionally performed using the FILE class. In the strict C sense, FILE is a structure and it is defined in the stdio.h header file. This object is equipped with variables used to indicate what operation would be performed. To use this structure, you can first declare an instance of the FILE structure. Here is an example:
FILE *Starter;
After instantiating this structure, you can define what to do with it, using one of the provided functions. Because FILE was created as a C structure, it does not have member functions. The functions used to perform its related operations on files are also declared in the stdio.h header file.
Opening and /or Saving Files
To create a new file, open an existing file, or save a file, you use the fopen() function. Its syntax is:
For example: FILE *fopen(“clients.dat”, “w”);
The first argument, FileName, must be a valid name of a file. If the user is creating or saving a new file, you can let him or her specify the name of the file, following the rules of the operating system. If the user is opening an existing file, you can make sure the file really exists, retrieve its name and pass it to the fopen() function.
Because the fopen() function is used to save a new file, to open an existing one, or to save a file that was only modified, the second argument, Mode, actually allows you to decide what operation the function will be used to perform. This argument is a short string of one or two characters and can be one of the following:
|
If the operation performed using the fopen() function is successful, the function returns a pointer to the FILE instance that was declared. COMMON PROGRAMMING ERRORS 1. Opening an existing file for writing (“w”) when, in fact, the user wants to preserve the file; the contents of the file are discarded without warning. What mode would you recommend? 2. Forgetting to open a file before attempting to reference it in a program. 3. Opening a non-existent file for reading. GOOD PROGRAMMING PRACTICE 1. Be sure that calls to file processing functions in a program contain the correct file pointers. 2. Explicitly close each file as soon as it is known that the program will not reference the file again. 3. Open a file only for reading and not update, if the contents of the file should not be modified. This prevents unintentional modification of the file’s contents. CONSIDER THE FOLLOWING PROGRAM. Its full explanation will be given in class. Remember to make note where necessary. |