When editing the file, the file should be in existence.
As a reminder, what we did was create a file in notepad as shown below and then populate it with information.
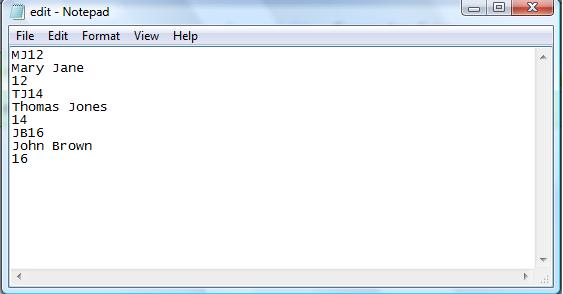
We then did the following:
- open the file, so that we could read the information from it into arrays.
- view the contents of the array to ensure that the correct data was in the array.
- ask the user to enter the id number of the record that needed to be edited.
- compare the id number entered by the user, with the idnums in the array
- if the id number exists, we ask what needs to be changed using a case structure and then allow the user to edit; if the id number does not exist we will inform the user.
- when the change is made, a view takes place to ensure the changes were made
- then we write to the file
Consider the program below:
#include<conio.h>
#include<stdio.h>
#include<string.h>
FILE *editptr;
int main()
{
char name[3][40], idnum[3][5], search[5];
int age[3];
if((editptr = fopen("edit.txt", "r")) == NULL)
{
printf("FILE CANNOT OPEN");
}
else
{
for(int record = 0; record < 3; record++)
{
fgets(idnum[record],5,editptr);
fscanf(editptr,"\n");//newline
fgets(name[record],40,editptr);
fscanf(editptr,"\n");//newline
fscanf(editptr, "%d", &age[record]);
fscanf(editptr,"\n");//newline
printf("Idnum is %s\nName is %sAge is %d\n\n",idnum[record],name[record],age[record]);
}
}
fclose(editptr);
printf("What is the id number for the record that needs to be changed?");
gets(search);
int position = 0;
int ans;
int choice;
while ((ans = strncmp(idnum[position], search, 4) != 0)&& (position<3))
{
position++;
}
if(position < 3)
{
printf("The record to be edited is %d", position);
printf("\n\nWhat would you like to edit?\n1. IDNUM\n2. Name\n3.AGE\n");
scanf("%d", &choice);
switch(choice)
{
case 1: printf("Enter new IDNUM\t");
fflush(stdin);
gets(idnum[position]);
break;
case 2: printf("Enter new Name\t");
fflush(stdin);
gets(name[position]);
break;
case 3: printf("Enter new age\t");
scanf("%d", &age[position]);
break;
default: printf("that option does not exist");
break;
}
}
else
{
printf("That ID number does not exist");
}
printf("\n\n\nThe updated records are:\n\n");
for(int record = 0; record < 3; record++)
{
printf("Idnum is %s\nName is %s\nAge is %d\n\n",idnum[record],name[record],age[record]);
}
//writing to the file
if((editptr = fopen("edit.txt", "w")) == NULL)
{
printf("FILE CANNOT OPEN");
}
else
{
for(int record = 0; record < 3; record++)
{
fprintf(editptr, "%s\n%s\n%d\n",idnum[record],name[record],age[record]);
}
}
fclose(editptr);
getch();
return 0;
}