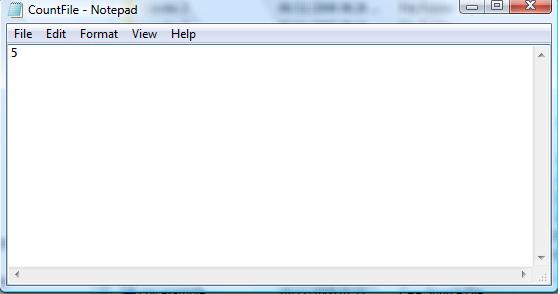
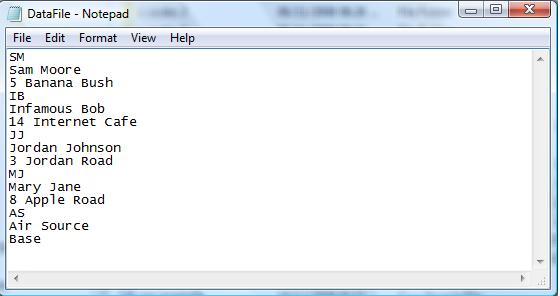
Consider the program below:
------------------------------------------------------------------------------------------------------------
#include<conio.h>
#include<stdio.h>
#include<string.h>
char name[100][50], address[100][75], idnum[100][3];
int count;
FILE *countptr;//pointer to the file that holds the next available position
FILE *dataptr; //pointer to the file that hold the records
void retrievecount();//stores the next available position in count and tells the number of records
void view ();//allow the user to view what is in the file
void deleterecord ();
int main()
{
view();
deleterecord ();
getch();
return 0;
}
void retrievecount()
{
//open the file to read the integer value in the file and store it in count.
if((countptr = fopen("CountFile.txt","r")) == NULL)
{
printf("File cannot open");
}
else
{
fscanf(countptr, "%d", &count);
}
fclose(countptr);
}
void view()
{
retrievecount();
if((dataptr = fopen("DataFile.txt","r")) == NULL)
{
printf("File cannot open");
}
else
{
//allow count to determine how many times you loop.
for (int c=0; c < count; c++)
{
fgets(idnum[c],3,dataptr);
fscanf(dataptr,"\n");//goes to next line
fgets(name[c],50,dataptr);
fscanf(dataptr,"\n");//goes to next line
fgets(address[c],75,dataptr);
fscanf(dataptr,"\n");//goes to next line
printf("ID num is %s.\nName is %sAddress is %s\n\n", idnum[c],name[c],address[c]);
}
}
fclose(dataptr);
}
void deleterecord ()
{
char delete_idnum [3];
retrievecount ();
printf("Please enter the id number to be deleted \t");
gets(delete_idnum);
if ((dataptr = fopen("DataFile.txt", "w"))== NULL)
{
printf("File cannot be opened");
}
else
{
for (int position =0; position < count; position++)
{
//re-writing to the file, all files except the one to be deleted
if(int ans = strcmp(delete_idnum, idnum[position])!= 0)
{
fprintf(dataptr,"%s\n", idnum[position]);
fprintf(dataptr,"%s", name[position]);
fprintf(dataptr,"%s",address[position]);
}
}
//updating count to reflect a lesser value
if((countptr = fopen("CountFile.txt","w"))== NULL)
{
printf("CountFile cannot open");
}
else
{
count = count-1;
fprintf(countptr,"%d",count);
}
fclose(countptr);
}
fclose(dataptr);
}
--------------------------------------------------------------------------------------------------------------
The changes will be reflected in the files:
- CountFile will have the number 4
- DataFile will have only 4 records.